

Notice that I didn't include 6 in the multiples of 3. We keep proceeding in this manner until the end. But remember the 4th index is already set to false since it was one of the multiples of 2. Again, the value in the 3rd index is TRUE and so we proceed in a similar manner setting all the multiple of 3 i.e. Hence we proceed to calculate its multiples i.e. Don't worry if this sounds confusing right now ( I'm not very good at explaining ) but this example will clear up everything - I promise!Įxample: We start with 2nd index of the array ( everytime). So what do we do once we find the multiples? We set the boolean value of that index to FALSE. If the value is TRUE, we calculate its multiple and if it's false we don't. But first we need to check if the current number ( or index of array ) is TRUE of false. Source: Step 3: Now starting from the 2nd index of the array to the last, we remove the multiples of the number. At first all of them are in the sieve machine (set to True) and then at last only the required particles (prime numbers) are present. It's analogous to how we filter out unwanted particles using a sieve. But as we proceed we'll have many False values. Step 2: Then all the elements of the array are set to boolean value TRUE. So now we have N-2 elements in the array. Step 1: We generate an array of numbers starting from 2 to N-1. Let's say we need to get all the prime numbers upto a number N. This algorithm cannot directly classify a number X as prime or composite but will generate list of all prime numbers that are less than X. The Sieve of Eratosthenes is a simple, ancient algorithm to find all the prime numbers that are less than a given number. This next algorithm gets the same result in just 50ms. But wait there's another even better algorithm for this type of prime calculations. Primality Test is in fact considered to be the fastest prime detection algorithm. Wot !! That's a massive performance gain if you ask me. # First algorithm : 705403.287ms // About 11 minutes # Using Primality algorithm : 117.574ms Hence there's much less computation to do. This algorithm does not give prime factors but only state whether the input number is prime or not. There's another much better way to classify a number as prime - the "Primality Test Algorithm". We have now significantly decreased the amount of divisors but this is still not good for large value of N. In our for loop in checkPrime function the loop should only continue up to √num. We can further optimize by taking divisors upto the square root of num. But that's still way off from being a good algorithm. That would decrease the processing time by half. By increasing the counter up by 2 in the two for loops we can ignore all the even numbers. We can optimize this by not classifying even numbers, except 2, since they are for sure not prime. In CS term the Big O of this approach is O(n 2) which is really really bad for an algorithm.

As the number increases, each classification will take more time than the previous one. To classify 13 we repeat the same process, divide it by every numbers starting from 2 to 12. To classify a prime number say 11, we divide it by every numbers starting from 2 upto 10. Lets see what's wrong with this approach.
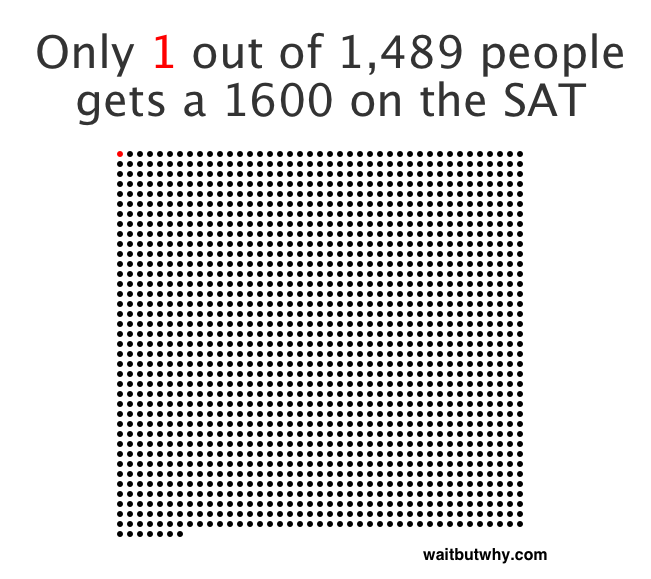
#List of prime numbers to 1000000 mod#
using the mod function in the where clauseĬonsole. cycle through all numbers and use LINQ to pull out the non-primes generate a list of numbers from 1 - 1,000,000

We only need to check divisors up until the square root of the last number in the set to cover all possible factors: We simply cycle through all the numbers from 1-1,000,000 and check to see if the number is divisible by the next possible factor. Or if the number has already been checked for all possible factors we keep it in the set.
#List of prime numbers to 1000000 code#
The heart of the code is just 4 lines of C# using LINQ. It uses a technique that continues to filter out factorable numbers from the set of all integers up to 1,000,000 until only the primes are left. This program takes advantage of the power of LINQ to generate all prime numbers from 1 to 1,000,000.
